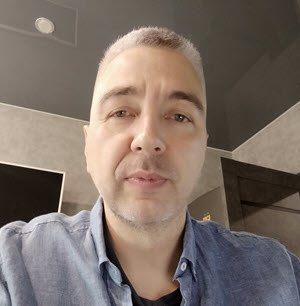
Vladimir Dyuzhev
MockMotor Creator
Creating a Simplest SOAP Service
Let's mock a single SOAP operation.
The fastest way to create a SOAP mock service is to import its WSDL.
But if we need to mock only a single operation of fifty, there is no need to complicate things. Let’s do it quickly.
Live Service: Canada Post’s Find Post Office
Canada Post has a simple SOAP web service that returns information about a post office.
The request looks like this:
POST https://ct.soa-gw.canadapost.ca/rs/soap/postoffice HTTP/1.1
Host: ct.soa-gw.canadapost.ca
SOAPAction: http://www.canadapost.ca/ws/soap/postoffice/GetPostOfficeDetail
Content-Type: text/xml
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:pos="http://www.canadapost.ca/ws/soap/postoffice">
<soapenv:Header/>
<soapenv:Body>
<pos:get-post-office-detail-request>
<locale>EN</locale>
<office-id>0000103157</office-id>
</pos:get-post-office-detail-request>
</soapenv:Body>
</soapenv:Envelope>
The key parameter is the office-id
.
The response contains a few data points about this post office:
HTTP/1.1 200 OK
Content-Type: text/xml
<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/">
<soap:Body>
<pos:get-post-office-detail-response xmlns:pos="http://www.canadapost.ca/ws/soap/postoffice">
<post-office-detail>
<address>
<city>TORONTO</city>
<latitude>43.77775</latitude>
<longitude>-79.34449</longitude>
<postal-code>M2J5A0</postal-code>
<province>ON</province>
<office-address>1800 SHEPPARD AVE E</office-address>
</address>
<location>BUCKINGHAM PO</location>
<name>DÉPANNEUR MAUZEROLL</name>
<office-id>0000103157</office-id>
<bilingual-designation>true</bilingual-designation>
<hours-list>
<day>1</day>
<time>09:00</time>
<time>17:00</time>
</hours-list>
<hours-list>
<day>2</day>
<time>09:00</time>
<time>17:00</time>
</hours-list>
<hours-list>
<day>3</day>
<time>09:00</time>
<time>17:00</time>
</hours-list>
<hours-list>
<day>4</day>
<time>09:00</time>
<time>17:00</time>
</hours-list>
<hours-list>
<day>5</day>
<time>09:00</time>
<time>14:00</time>
</hours-list>
</post-office-detail>
</pos:get-post-office-detail-response>
</soap:Body>
</soap:Envelope>
This is all we need to know to mock this transaction.
Let’s do it!
Step 1. (Optional) Create a Mock Environment
In most cases, we only add mock services to existing environments. But for the sake of completeness, let’s create a dedicated mock environment.
1 Click on the Mock Environments
link at the top left part of the screen to navigate to the Environments page.
2 Click on the Add Environment
button to create a new environment.
3 Name the environment appropriately. In my case, Provisioning
.
4 Save it. The ports will be assigned automatically.
Step 2. Create Post Office Mock Service
If you just created a mock environment, you’re already within it. If you did not, navigate to your environment of choice
by clicking on the Mock Environments
link at the top left part of the screen, then finding
the desired environment in the list and clicking on it.
1 Click on the Services
tab.
2 Click on the Add Service
button.
3 Name the service Post Office
and provide the prefix URL of /rs/soap/postoffice
.
4 Save the service.
You can notice that the Service URL
field is now showing an URL like http://127.0.0.1:20010/rs/soap/postoffice
- this is the URL of your new mock service.
Note it contains the environment port 20010
followed by the service prefix /rs/soap/postoffice
.
Step 3. Create the Reaction
Now let’s add a mock reaction for the get-post-office-detail-request
request.
Making an Empty Response
1 Navigate to the Reactions
tab within the Post Office
service.
2 Click Add Reaction
and select Manually...
from the dropdown.
Setting General Properties
A new reaction page will open.
3 Enter BUCKINGHAM PO
as the name for the new response.
4 Since the response payload is SOAP, set the scripting language to XQuery. We do not use scripting in this static response, but if we later add any conditional logic, it will be executed with the XQ engine.
Setting Match Options
5 The request is using HTTP POST, so make sure the match method to POST
.
6 Set SOAP Action
to http://www.canadapost.ca/ws/soap/postoffice/GetPostOfficeDetail
.
6* Alternatively, set First Element of XML Payload
to get-post-office-detail-request
. The first element of the payload is the first element under the SOAP Body element.
7 To match only requests with specific office-id
, enter the XQuery match script: $input//*:office-id='0000103157'
When an HTTP POST for URL
http://127.0.0.1:20000/rs/soap/postoffice
comes in, MockMotor has to select the environment, service and reaction to handle this request.Environment: Our
Post Office
environment listens on port20000
. Because of this match with the URL port, MockMotor routes the request to this environment.Service: MockMotor then decides what service in the environment should handle the request. The
/rs/soap/postoffice
part of the URL matches thePost Office
service’s prefix, and so the request is routed to that service.Reaction: MockMotor reviews all reactions within the
Post Office
service until it finds one that has a full match in theMatch Options
section. Our mock reaction says it must match by HTTP methodPOST
(it does) and by SOAP Action relative URLhttp://www.canadapost.ca/ws/soap/postoffice/GetPostOfficeDetail
(it does).Then the match script is executed if provided. The match script finds the
office-id
value in the request and compares it with0000103157
. It matches.This reaction hence is selected and executed.
Setting Reaction Payload
8 Place the static response into the Payload
field.
9 Since the response is SOAP, set the Content-Type
field to text/xml
.
10 Save the reaction.
Reviewing the Result
Under the Reactions
tab, you can now see the newly created reaction with its summary - name, HTTP method, matching options and other details.
Step 4. Try it
Our new service is ready and listening on http://127.0.0.1:20010/rs/soap/postoffice
(and on HTTPS at 20011
).
Since the operation uses HTTP POST, we have to call it using SoapUI or Postman.