Quick Start: Creating Data-Driven Service
How to make the same response provide different data depending on the request content?
Of course you can have a separate response for each request, but that is not scalable - what if you need 1000 responses? 10000 responses?
MockMotor supports something called mock accounts that approximates (mocks?) real accounts of the backend system. A mock account can be selected based on the request data (say account number) and the response can be generated with mock account values in it.
Let’s see how we can do that.
Canada Post PostOffice Service
I will use Canada PostOffice SOAP service as the example. It only has two operations, and we’re going to make GetPostOfficeDetail
a data-driven one.
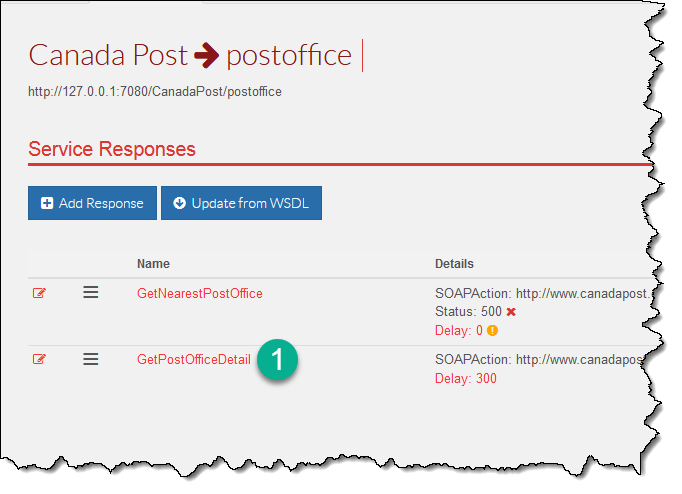
Originally, I had a hardcoded response for GetPostOfficeDetails
, i.e. it always returned the same address:
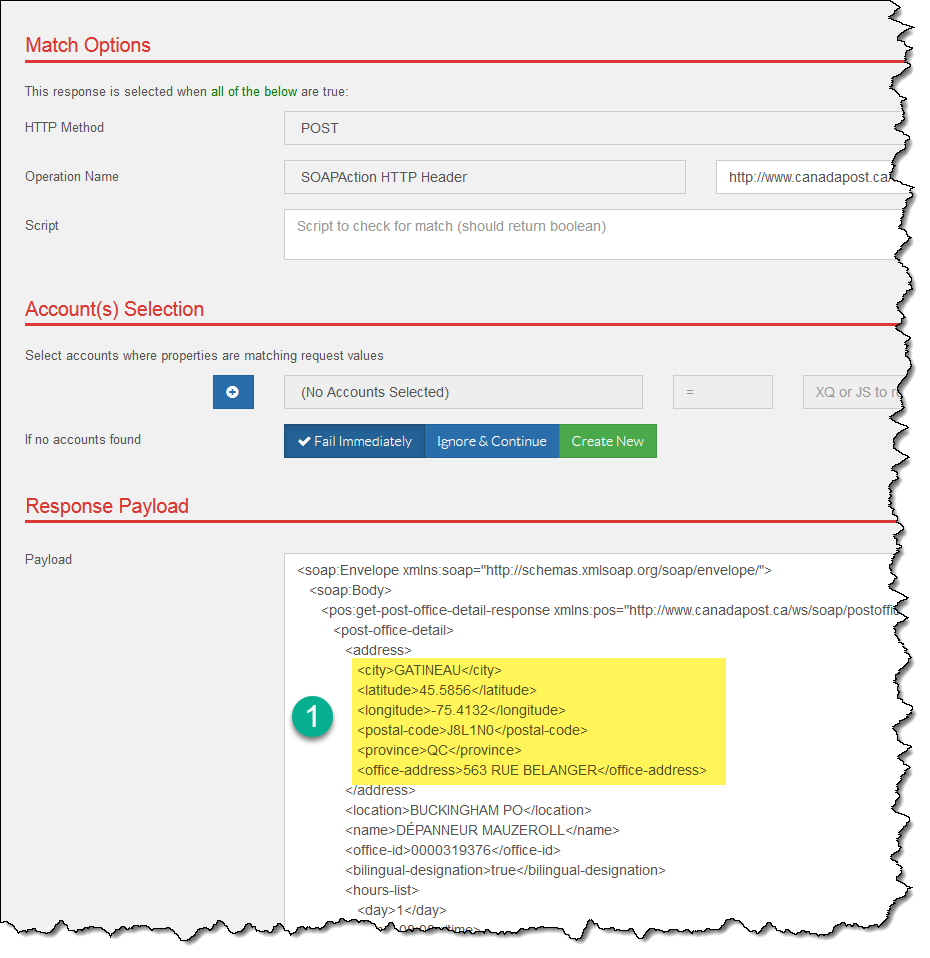
Call for Data-Driven
But then QA folks need to test more scenarios. For instance, they need an address in Ontario, and another one in Quebec, and probably 100 other variations.
We do not want to do 100 variations of hardcoded responses, and so it is time to use mock accounts.
Take a look at the part of the response that we need to modify:
<address>
<city>GATINEAU</city>
<latitude>45.5856</latitude>
<longitude>-75.4132</longitude>
<postal-code>J8L1N0</postal-code>
<province>QC</province>
<office-address>563 RUE BELANGER</office-address>
</address>
```
We have properties `city`, `latitude`, `longitude`, `postal-code`, `province` and `office-address`. These are the properties that we need to script based on a mock account.
It may help to think of these properties as columns in a mock accounts spreadsheet.
We also need to add `office-id` property that comes in the request, because this is how we're going to select the account used in the response:
<soapenv:Envelope xmlns:soapenv=“http://schemas.xmlsoap.org/soap/envelope/" xmlns:pos=“http://www.canadapost.ca/ws/soap/postoffice">
soapenv:Header/
soapenv:Body
pos:get-post-office-detail-request
## Creating Mock Account Properties
Mock accounts are environment-wide, and so account properties are configured on environment level.
Navigate to environment Accounts tab, and then to Account Properties.
You can see that the list of properties is currently empty. Not for long!
Click on Add Property to add a new property:
A property has a mandatory name which is name used in scripts, and a few other optional values.
Here I create a property `city` with a human-readable name `City` (that was unnecessary, really; human-readable name is for cases when the property name along doesn't explain its purpose).
Then I click on Save to, well, _save_ the property:
Repeating this operation for each of our modifiable values, we get a list of all properties:
Property name cannot have anything except alphanumeric symbols, so the dashes had to be removed.
## Update Response
Now lets navigate back to GetPostOfficeDetail response and make it select and use an account based on the request.
### Select Account
The request, to remind, has an `office-id` value:
pos:get-post-office-detail-request
We're going to use that value to select a mock account.
Scroll down to Accounts Selection section. Currently it selects no accounts. Click on plus sign to add a selection condition:
From the drop-down, select the account property (`officeId`) we'll be comparing the request value with. The request value is extracted with XPath `$input//*:office-id`:
MockMotor will execute the XPath to retrieve the request value, and then find all the environment mock accounts that have that value in `officeId` property.
The found account is placed into `$account` (and `$accounts` if many found) variable.
If such an account is not found, the request will fail immediately.
### Update Response Payload
Now let's modify the payload to take the values from the `$account` variable. Every mock account property is accessible with `$account//*:propertyName/text()` XPath syntax:
All other values in the response remain hardcoded. Since we do not have test scenarios that could be affected by that, we do not really care.
### Save Response
Finally, save the response:
## Account Not Found Case
Now every request to GetPostOfficeDetail fails because it looks for a mock account, but we did not create any accounts yet:
You see that MockMotor actually tries to use the office-id value to search for accounts, but alas, none found.
Good, we have a baseline.
## Creating Accounts with Excel
The fastest way to create _a lot_ of accounts at once is to upload them with an Excel spreadsheet.
Let's navigate back to Accounts tab and click on Download as XLS:
It'll download an Excel file which is currently empty (no accounts) except for column names (property names):
Save this file and modify it any way you like - just keep the header names in place.
I have created two mock accounts - one for a Quebec post office, and one for an Ontario one:
Now upload this file back to MockMotor: click on Replace Accounts from XLS:
In the appeared dialog select the updated spreadsheet location and click Import & Replace:
The accounts from Excel are imported into the environment:
## Test the Response Generation
Now lets try to execute requests to GetPostOfficeDetail with `office-id` matching our mock accounts (officeId 102978 and 103157):
First account (QC):
Second account (ON):
As you can see, the values in the response are populated from the selected account.
## Tip: Check the Execution Log
To help in troubleshooting, MockMotor reports what account was used for an invocation in the execution log.