JWT (JSON Web Tokens)
MockMotor implements JWT support natively.
Every reaction with Javascript has access to JWT library. That library is capable of generating, parsing and verifying JWT tokens.
XQuery-based reactions don't have JWT support yet. If you need it, please let me know.
MockMotor JWT API
JWT functions can be used in any reaction’s scriptable field.
Function | Purpose | Result | Example |
---|---|---|---|
JWT.encode() | Creates a JWT token from a header, payload and a secret. Takes the signature algorithm from the header’s alg field. Currently only HS256 , HS384 and HS512 are supported. |
JWT token as string | var token = JWT.encode(header,payload,"password123"); |
JWT.decode() | Decodes a JWT token into JSON format. The operation doesn’t fail if the token is invalid, but it adds an error field to the resulting object. |
JSON with a header , payload and optionally error fields. |
var jwt = JWT.decode(JWT.token,"password123"); |
JWT.verify() | Decodes and verifies JWT token into JSON format. Currently only HS256 , HS384 and HS512 algorithms are supported. In case of the invalid token or an unsupported algorithm, the reaction fails with a hard error (HTTP 500). |
JSON with a header and payload fields. |
var jwt = JWT.verify(JWT.token,"password123"); |
JWT.token | Contains JWT token as a string taken from Authorization: Bearer header or null. |
JWT Token | if( JWT.token ){ ... } |
JWT.header | Contains JWT header as a JSON object taken from Authorization: Bearer header or empty object. |
JWT header | var issuer = JWT.header.iss; |
JWT.payload | Contains JWT payload as a JSON object taken from Authorization: Bearer header or empty object. |
JWT payload | var userId = JWT.payload.user; |
If the request’s Authorization
header doesn’t have a JWT, the JWT.token
, JWT.header
and JWT.payload
are null
.
Example: Generating a JWT
To implement a reaction that generates a JWT, we need to form JWT header and payload, and then sign it with a secret value (not-so-secret in this case - it’s just a mock!).
Look at this payload script:
var header = {
"alg": "HS256",
};
var payload = {
"userid": "john.doe"
};
var token = JWT.encode(header,payload,"qwerty");
output = {"token":token};
The response contains the generated JWT, like below:
{
"token": "eyJhbGciOiJIUzI1NiJ9.eyJ1c2VyaWQiOiJqb2huLmRvZSJ9.CuScq77_iCP4XsYGCMgGnQiATOmQwu_rR1LEB2Pcd_I"
}
The function takes the signature algorithm from the header’s alg
value, HS256
in this case.
(You can decode and verify this token at Auth0 JWT site.)
Example: Decoding JWT to Get User Login
Suppose we need to select a mock account based on login
value passed in JWT payload.
We can use JWT.payload.login
in the account selection. Before the reaction execution begins, MockMotor decodes the request’s Authorization: Bearer
header into the JWT.payload
JSON object, so you don’t need to decode it yourself.

Example: Verifying JWT is Valid
Sometimes you need to check if the passed JWT is valid.
For example, you may want to properly mock the exp
(Expiration Time Claim) or nbf
(Not Before Claim),
and reject a request when the JWT is not yet valid or has already expired.
To verify a token a secret is required, and so MockMotor can’t do that automatically. You need to call
to JWT.decode()
or JWT.verify()
in a reaction’s scripted field.
For example, here I implemented a “JWT Check” - a reaction that matches only when the token is invalid.
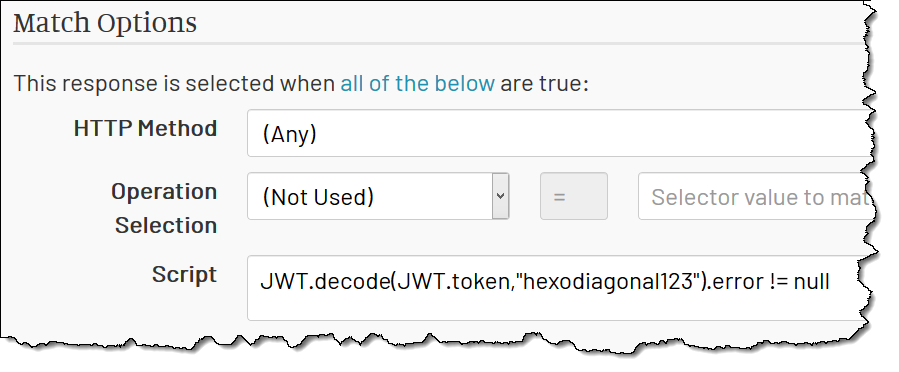
The JTW.decode()
function takes the token JWT.token
and a secret (password) and decodes the token into a JSON structure. However, if the token is invalid, it
also adds an error
field to that structure. If the reaction sees the error
present (not null), it matches the request. This response has a status HTTP 401,
effectively rejecting the call.
If, on the other hand, the token is valid, the reaction doesn’t match and is passed down to the next reaction which now doesn’t have to worry about the JWT validity.